Algo Expert Cube Clone
Inspired by AlgoExpert website UI
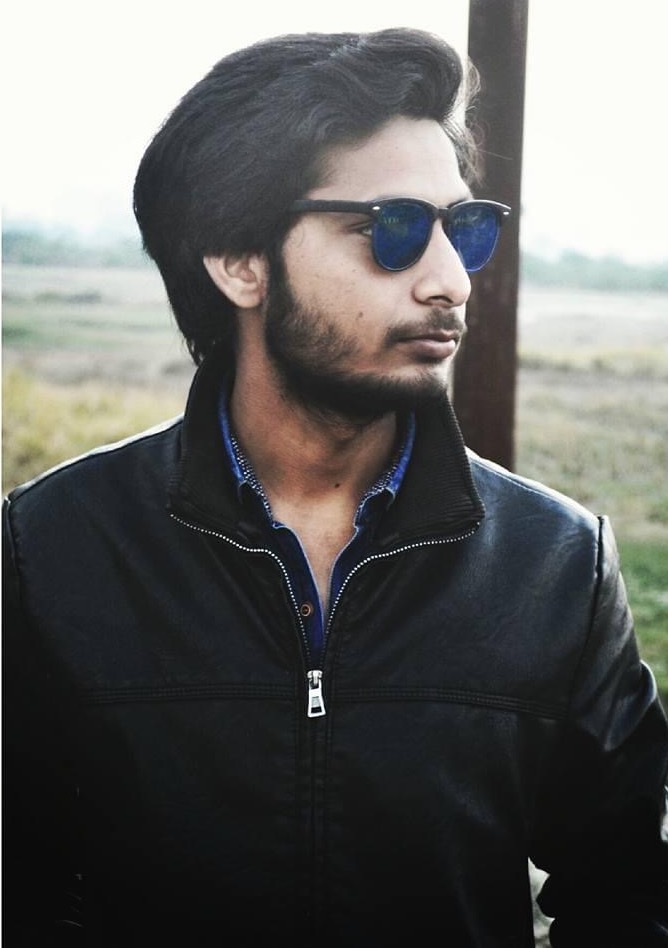
Suboor Khan
Software Engineer
There's nothing more frustrating than opening an interview prep book, only to find a bunch of solutions in a programming language that you don't know. That's why all of our questions come with complete written solutions in 9 popular languages.
How did i make it?
Index.HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>
CodePen - Basic Three.js Example</title>
<link rel="stylesheet" href="./style.css">
<link rel="stylesheet"
href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0/css/bootstrap.min.css"
integrity="sha384-Gn5384xqQ1aoWXA+058RXPxPg6fy4IWvTNh0E263XmFcJlSAwiGgFAW/dAiS6JXm"
crossorigin="anonymous">
</head>
<body>
<div class="container mt">
<div id="cube">
</div>
<p>
<b>
There's nothing more frustrating than opening an interview prep book, only to find a
bunch of solutions in a programming language that you don't know. That's why all of our questions
come with complete written solutions in 9 popular languages. </b>
</p>
</div>
<script src='https://threejs.org/build/three.js'>
</script>
<script src="./script.js">
</script>
</body>
</html>
style.css
canvas { width: auto !important; height: 550px !important }
#cube {
max-width: 100px !important;
}
script.js
let camera, scene, renderer, cube;
function init() {
// Init scene
scene = new THREE.Scene();
// Init camera (PerspectiveCamera)
camera = new THREE.PerspectiveCamera(
75,
window.innerWidth / window.innerHeight,
0.1,
1000
);
// Init renderer
renderer = new THREE.WebGLRenderer({ antialias: true });
console.log(renderer);
renderer.setClearColor ( "white", 1 )
// Set size (whole window)
renderer.setSize(window.innerWidth, window.innerHeight);
// Render to canvas element
document.getElementById("cube").appendChild(renderer.domElement);
// Init BoxGeometry object (rectangular cuboid)
const geometry = new THREE.BoxGeometry(3, 3, 3);
// Create material with color
// const material = new THREE.MeshBasicMaterial({ color: 0x0000ff });
// Add texture -
const texture = new THREE.TextureLoader().load('img/react.png');
// Create material with texture
var materialArray = [];
materialArray.push(new THREE.MeshBasicMaterial( { map: THREE.ImageUtils.loadTexture('img/react.png' ), side: THREE.DoubleSide, transparent: true, opacity: 0.9 }));
materialArray.push(new THREE.MeshBasicMaterial( { map: THREE.ImageUtils.loadTexture( 'img/angular.png' ), side: THREE.DoubleSide, transparent: true, opacity: 0.9 }));
materialArray.push(new THREE.MeshBasicMaterial( { map: THREE.ImageUtils.loadTexture('img/vue.jpeg' ), side: THREE.DoubleSide, transparent: true, opacity: 0.9 }));
materialArray.push(new THREE.MeshBasicMaterial( { map: THREE.ImageUtils.loadTexture( 'img/laravel.jpeg' ), side: THREE.DoubleSide, transparent: true, opacity: 0.9 }));
materialArray.push(new THREE.MeshBasicMaterial( { map: THREE.ImageUtils.loadTexture('img/go.png' ), side: THREE.DoubleSide, transparent: true, opacity: 0.9 }));
materialArray.push(new THREE.MeshBasicMaterial( { map: THREE.ImageUtils.loadTexture( 'img/node.png' ), side: THREE.DoubleSide, transparent: true, opacity: 0.9 }));
// Create mesh with geo and material
cube = new THREE.Mesh(geometry, materialArray);
// Add to scene
scene.add(cube);
// Position camera
camera.position.z = 5;
}
// Draw the scene every time the screen is refreshed
function animate() {
requestAnimationFrame(animate);
// Rotate cube (Change values to change speed)
cube.rotation.y += 0.01;
cube.rotation.x = 0.1;
renderer.render(scene, camera);
}
function onWindowResize() {
// Camera frustum aspect ratio
camera.aspect = window.innerWidth / window.innerHeight;
// After making changes to aspect
camera.updateProjectionMatrix();
// Reset size
renderer.setSize(window.innerWidth, window.innerHeight);
}
window.addEventListener('resize', onWindowResize, false);
init();
animate();