Highcharts in React
Highcharts is a modern SVG-based, multi-platform charting library. It makes it easy to add interactive charts to web and mobile projects. It has been in active development since 2009, and remains a developer favorite due to its robust feature set, ease of use and thorough documentation.
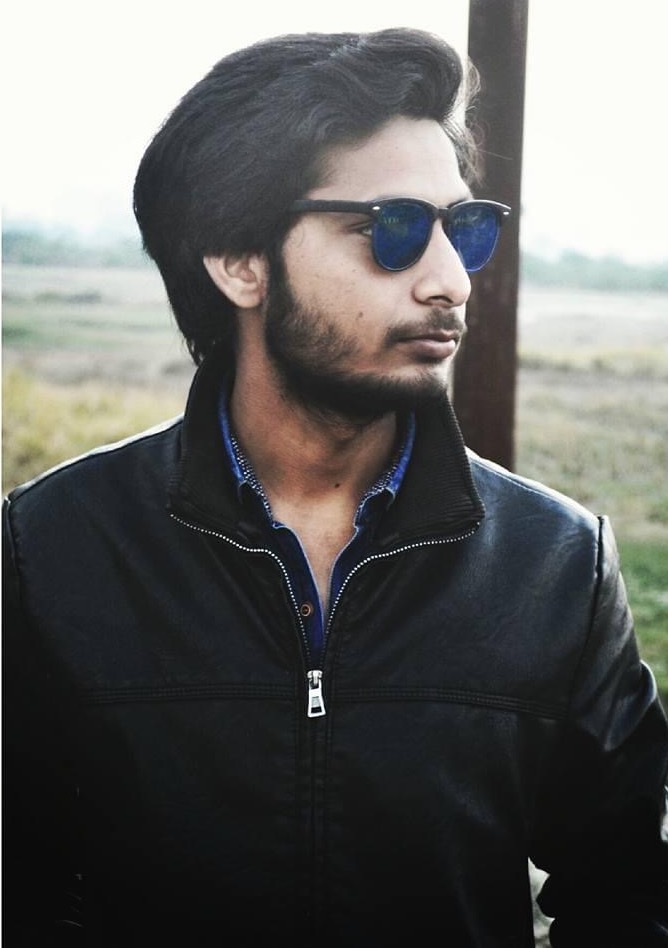
Suboor Khan
Software Engineer
Home.jsx
import React, { Component } from "react";
import { render } from "react-dom";
// Import Highcharts
import Highcharts from "highcharts/highstock";
// Import our demo components
import Chart from "./chart.jsx";
// Load Highcharts modules
require("highcharts/indicators/indicators")(Highcharts);
require("highcharts/indicators/pivot-points")(Highcharts);
require("highcharts/indicators/macd")(Highcharts);
require("highcharts/modules/exporting")(Highcharts);
require("highcharts/modules/map")(Highcharts);
const chartOptions = {
title: {
text: "",
},
series: [
{
data: [1, 2, 3],
},
],
};
export default class Home extends Component {
constructor(props) {
super(props);
this.state = {
chartConfig: {
options: {
title: {
text: "Solar Employment Growth by Sector, 2010-2016",
},
subtitle: {
text: "Source: thesolarfoundation.com",
},
yAxis: {
title: {
text: "Number of Employees",
},
},
xAxis: {
accessibility: {
rangeDescription: "Range: 2010 to 2017",
},
},
legend: {
layout: "vertical",
align: "right",
verticalAlign: "middle",
},
plotOptions: {
series: {
label: {
connectorAllowed: false,
},
pointStart: 2010,
},
},
series: [
{
name: "Installation",
data: [43934, 52503, 57177, 69658, 97031, 119931, 137133, 154175],
},
{
name: "Manufacturing",
data: [24916, 24064, 29742, 29851, 32490, 30282, 38121, 40434],
},
{
name: "Sales & Distribution",
data: [11744, 17722, 16005, 19771, 20185, 24377, 32147, 39387],
},
{
name: "Project Development",
data: [null, null, 7988, 12169, 15112, 22452, 34400, 34227],
},
{
name: "Other",
data: [12908, 5948, 8105, 11248, 8989, 11816, 18274, 18111],
},
],
responsive: {
rules: [
{
condition: {
maxWidth: 500,
},
chartOptions: {
legend: {
layout: "horizontal",
align: "center",
verticalAlign: "bottom",
},
},
},
],
},
},
},
};
}
render() {
return (
);
}
}
//Chart.js
import React from 'react'
import HighchartsReact from 'highcharts-react-official'
const Chart = ({ options, highcharts }) => <HighchartsReact
highcharts={highcharts}
constructorType={'chart'}
options={options}
/>
export default Chart